Lab Topic 12 individual
.docx
keyboard_arrow_up
School
Texas A&M University *
*We aren’t endorsed by this school
Course
102
Subject
Computer Science
Date
Jan 9, 2024
Type
docx
Pages
2
Uploaded by ProfLightning103643 on coursehero.com
Lab: Topic 12 (individual)
ENGR 102
Lab: Topic 12 (individual)
Deliverables
:
There are two deliverables for this assignment. Please submit the following files to zyBooks:
●
pretty_plot.py
●
plotting_data.py
This week’s team and individual assignments are meant to familiarize you with two of the most
commonly used engineering packages in Python:
numpy
and
matplotlib
. This individual assignment
is meant to follow on to the team lab work.
It is best to complete the team lab first.
Activity #1
: Pretty plot – individual
Create a program named
pretty_plot.py
that repeatedly multiplies a matrix by a point and plots the
results.
Start with a 2D point,
(
x, y
)
. This point can be represented as a vector:
v
=
[
x y
]
. There is
also defined a 2x2 matrix,
M
=
[
abc d
]
. Computing the product of
M
with
v
will give
a new point
v '
:
v
'
=
Mv
. Then, multiply the matrix
M
by the new point
v '
, to get
another point, i.e.
v
' '
=
M v
'
. This can go on indefinitely, creating a long sequence of points.
Your program should use
numpy
to create a matrix and a point. Begin with the point
(
0,1
)
and the
matrix:
[
1.010.09
−
0.091.01
]
. Then, multiply the matrix by the point to get a new point. Repeat for
a total of 200 times. Have your program plot the data points using
matplotlib
. Be sure to label the x
and y axes, and include a title. Your title should give a brief description of the shape that the points
“trace” out.
Note
: the purpose of this activity is to get practice with
numpy
, so you should use
numpy
for your
operations, even if you find it easier to perform this computation a different way.
Activity #2
: Plotting data – individual
This program will be a follow-on to the program from last week’s individual assignment, Activity #3. In
that assignment, you read in weather data from the file
WeatherDataCLL.csv
.
Write a program named
plotting_data.py
to (again) read in weather data from the same file and plot the
data in a set of graphs described below. You may re-use code from the previous assignment for reading
in data from a file. Using
matplotlib
, create the following 4 graphs.
1)
Create a
line graph
that shows both the maximum temperature and average wind speed plotted
over the period of time. Both lines should be plotted on the same graph, with date on the x-axis,
and
different
y axes for the two different measurements.
(Please do not spend time dealing with
“date data type.” Please just consider the dates in the data to be strings, or, you may simply
consider the days as integers for plotting.)
2)
Create a
histogram
of the average wind speed. The x axis should cover a reasonable range of
average wind speeds, and the y axis should show the number of days that had an average wind
speed in the specific range.
3)
Create a
scatterplot
indicating the relationship (or lack thereof) between average wind speed
and minimum temperature (one on each axis).
Based upon Dr. Keyser’s Original1Revised Summer 2023 JL
Lab: Topic 12 (individual)
4)
Create a
bar chart
, with one bar per calendar month (each month from all 3 years), showing the
average temperature, along with lines indicating the highest high and lowest low temperatures
from that month.
a.
Note: You may want to create new lists of data, but you may find it useful to use the
max/min/sum functions on lists.
b.
This is a great problem to practice using dictionaries!
See the example plots below. Your plots do
NOT
have to look exactly the same, but they
must
include a
title, axis labels, and a legend (where appropriate). You should also learn how to control the width, color,
and shape of your lines and plotted points, as well as ensure your scales/ranges are set appropriately to
display all of the data nicely, however these are highly subjective and you will not be graded on them.
Include all four (4) plots in your program.
Based upon Dr. Keyser’s Original2Revised Summer 2023 JL
Plot 1
Plot 2
Plot 3
Plot 4
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
describe the code in detail add comment lines
package com.templatepattern;
public class TemplatePatternTest1 {
public static void main(String[] args) {String connectionString = "server=192.168.0.7";String username = "learner";String password = "designPatterns";StudentRegistration sr = new DatabaseBasedStudentRegistration(connectionString, username, password);System.out.println();Person p1 = new Person("patrick", "nick");System.out.println("Registering " + p1);sr.register(p1);System.out.println();
Person p2 = new Person("zayn", "nick");System.out.println("Registering " + p2);sr.register(p2);System.out.println();}
}
abstract class StudentRegistration {// template methodfinal Student register(Person person) {if (checkPerson(person)) {startOperation();try {int studentId = getNewIdentity(person);Student student = new Student(person, studentId);completeOperation();return student;}catch (Exception e) {cancelOperation();promptError(e.getMessage());}}else {promptError(person + " is not a valid…
arrow_forward
/** To change this license header, choose License Headers in Project Properties.* To change this template file, choose Tools | Templates* and open the template in the editor.*/package Apps;
import ADTs.QueueADT;import DataStructures.ArrayStack;import ADTs.StackADT;import DataStructures.LinkedQueue;import java.util.Scanner;
/**** @author Qiong*/public class RepeatStrings {public static void main(String[] argv) throws Exception{final int SIZE = 3;Scanner keyboard = new Scanner(System.in);QueueADT<String> stringQueue;//stringQueue = new CircularArrayQueue<String>(SIZE);stringQueue = new LinkedQueue<String>();StackADT<String> stringStack;stringStack = new ArrayStack<String>(SIZE);String line;for (int i = 0; i < SIZE; i++){System.out.print("Enter a line of text which includes only 3 words > ");line = keyboard.nextLine();//TODO enque the new element//TODO push the new element}System.out.println("\nOrder is: ");for (int i = 0; i < SIZE; i++){// TODO…
arrow_forward
1. A list of students (student ID, first name, last name, list of 4 test grades) will be transformed intoStudent objects. We will provide this file for you.a. Each feature will be separated by commas, however; the grades will be separated byspaces, as shown in this example line: 82417619,Erik,Macik,95.6 85 100 88[Hint: It seems like you might need to use the split method twice]b. Your program should work with any file with any number of lines.2. A menu will be displayed on loop to the user (meaning after a user completes a selection, themenu will be displayed again, unless the user exits).The menu options should be the following:a. View Student Grade and Averageb. Get Test Averagec. Get Top Student per examd. Exit3. Your system shall incorporate the following classes. Each class will require one file.GRADE CALCULATORPurposeA class that contains useful methods that can be used to calculate averages andconvert grades to letter gradesAttributes: NoneMethodsconvertToLetterGrade(double…
arrow_forward
A document is represented as a collection paragraphs, a paragraph is represented as a collection of sentences, a sentence is represented as a collection of words and a word is represented as a collection of lower-case ([a-z]) and upper-case ([A-Z]) English characters.
You will convert a raw text document into its component paragraphs, sentences and words. To test your results, queries will ask you to return a specific paragraph, sentence or word as described below.
Alicia is studying the C programming language at the University of Dunkirk and she represents the words, sentences, paragraphs, and documents using pointers:
A word is described by .
A sentence is described by . The words in the sentence are separated by one space (" "). The last word does not end with a space(" ").
A paragraph is described by . The sentences in the paragraph are separated by one period (".").
A document is described by . The paragraphs in the document are separated by one newline("\n"). The last paragraph…
arrow_forward
A document is represented as a collection paragraphs, a paragraph is represented as a collection of sentences, a sentence is represented as a collection of words and a word is represented as a collection of lower-case ([a-z]) and upper-case ([A-Z]) English characters.
You will convert a raw text document into its component paragraphs, sentences and words. To test your results, queries will ask you to return a specific paragraph, sentence or word as described below.
Alicia is studying the C programming language at the University of Dunkirk and she represents the words, sentences, paragraphs, and documents using pointers:
A word is described by .
A sentence is described by . The words in the sentence are separated by one space (" "). The last word does not end with a space(" ").
A paragraph is described by . The sentences in the paragraph are separated by one period (".").
A document is described by . The paragraphs in the document are separated by one newline("\n"). The last paragraph…
arrow_forward
m6 lb
Dont copy previous answers they are incorrect. Please provide a typed code and not pictures. The template was given below the code. we are just making changes to main cpp. We are writing one line of code based on the comments.
C++
use shared pointers to objects, create vectors of shared pointers to objects and pass these as parameters.
You need to write one line of code. Declare circleTwoPtr. Watch for **** in comments.
Template code is given make changes and add stuff based on comments:
Template given:
main.cpp:
#include<iostream>#include "Circle.h"#include <fstream>#include <vector>#include <memory>#include<cstdlib>#include <fstream>#include <sstream>using namespace std;const int SIZE = 10;// pay attention to this parameter listint inputData(vector<shared_ptr<Circle>> &circlePointerArray, string filename)
{ ifstream inputFile(filename); istringstream instream; string data; int count =0; int x,y,radius; try{ if…
arrow_forward
Hey, question about DLL-libraries, C#.
So I did a project where I have two classes database.cs & info.cs. I created .dll file about those in other project. Now I added .dll file to my main project to References and it's there. I'm using "using ClassMembers(dll file) in other forms now. Do I delete my two classes database.cs & info.cs now or do I keep them? This is my first time using .dll files and I'm kinda confused, what to do now..
arrow_forward
Building Additional Pages
In this lab we will start to build out the Learning Log project. We’ll build two pages that display data: a page that lists all topics and a page that shows all the entries for a particular topic. For each of these pages, we’ll specify a URL pattern, write a view function, and write a template. But before we do this, we’ll create a base template that all templates in the project can inherit from.
arrow_forward
Considering the dates API in java.time, it is correct to say:
1.
It is possible to represent a date as text in any language, as long as you provide the respective information as Locale.
B.
It is not a Postgres database compatible type.
Ç.
It is not possible to represent dates before the year 2000.
D.
The main class in this package is java.util.Date.
5.
It follows the standard defined in ISO 8601, so it is ONLY possible to display the data in English.
arrow_forward
Need help with this python question. You can't use break, str.endswith, list.index, keywords like: await, as, assert, class, except, lambda, and built in functions like: any, all, breakpoint, callable.
(Question in images at bottom)
Starter Code
import random
def spider_web(web_map, starting_place, destination):
pass
def spider_web_rec(web_map, starting_place, destination, visited):
pass
def make_spider_web(num_nodes, seed=0):
if seed:
random.seed(seed)
web_map = {}
for i in range(1, num_nodes + 1):
web_map[f'Node {i}'] = []
for i in range(1, num_nodes + 1):
sample = random.sample(list(range(i, num_nodes + 1)), random.randint(1, num_nodes - i + 1))
print('sample', i, sample)
for x in sample:
if i != x:
web_map[f'Node {i}'].append(f'Node {x}')
web_map[f'Node {x}'].append(f'Node {i}')
return web_map
if __name__ == '__main__':
num_nodes, seed = [int(x) for x…
arrow_forward
hello c++ programming
Superhero battle time! Muscles and Bones are not happy with each other and need to come to some kind of understandingWhat are the odds that Muscles will come out ahead? What about Bones?
Start with this runner file that will handle the simulation, and don't modify the file in any way (runner file is below)Spoiler time... When it's all said and done, Muscles should win about 72% of the time, and Bones about 28%
Create files TwoAttackSupe.h and TwoAttackSupe.cpp that collectively contain the following functionality:
The class name will be TwoAttackSupe
The class will be a base class for what you see below in the next blurb
The class has one member variable, an integer value that maintains a health value
Its only constructor takes one parameter, which is the health value
Make sure to set up a virtual destructor since this is a base class
Develop a function called IsDefeated() which returns a bool
Return true if the health value is less than or equal to 0
Return…
arrow_forward
You must convert this C file into C++ environments without using Classes conceptCODE: CONTACT MANAGEMENT SYSTEM#include<stdio.h>#include<conio.h>#include<string.h>#include<process.h>#include<stdlib.h>#include<dos.h>struct contact{ long ph; char name[20],add[20],email[30];} list;char query[20],name[20];FILE *fp, *ft;int i,n,ch,l,found;int main(){main: system("cls"); /* ************Main menu*********************** */ printf("\n\t **** Welcome to Contact Management System ****"); printf("\n\n\n\t\t\tMAINMENU\n\t\t=====================\n\t\t[1] Add a new Contact\n\t\t[2]List all Contacts\n\t\t[3] Search for contact\n\t\t[4] Edit aContact\n\t\t[5] Delete a Contact\n\t\t[0]Exit\n\t\t=================\n\t\t"); printf("Enter the choice:"); scanf("%d",&ch); switch(ch) { case 0: printf("\n\n\t\tAre you sure you want to exit?"); break; /* *********************Add new contacts************ */ case 1: system("cls"); fp=fopen("contact.dll","a"); for (;;) {…
arrow_forward
Please written by computer source
Assignment 4 In this assignment you will be using the dataset released by The Department of Transportation. This dataset lists flights that occurred in 2015, along with other information such as delays, flight time etc.
In this assignment, you will be showing good practices to manipulate data using Python's most popular libraries to accomplish the following:
cleaning data with pandas make specific changes with numpy handling date-related values with datetime Note: please consider the flights departing from BOS, JFK, SFO and LAX.
Each question is equally weighted for the total grade.
import os
import pandas as pd
import pandas.api.types as ptypes
import numpy as np
import datetime as dt
airlines_df= pd.read_csv('assets\airlines.csv')
airports_df = pd.read_csv('assets\airports.csv')
flights_df_raw = pd.read_csv('assets\flights.csv', low_memory = False)
Question 1: Data Preprocessing
For this question, perform the following:
remove rows with…
arrow_forward
Python IDlE: I get the below error: How can I adjust my code?
Traceback (most recent call last): File "C:\Users\16304\OneDrive\Documents\Homework\practice2_2.py", line 22, in <module> piggyBank(["Q"]) File "C:\Users\16304\OneDrive\Documents\Homework\practice2_2.py", line 20, in piggyBank value = (plist + nlist + dlist+ qlist)UnboundLocalError: local variable 'dlist' referenced before assignment
Function Below:
def piggyBank(list): typelist =["Q","D","N","P"] for i in list: if "Q" in typelist: qlist =(list.count("Q")*25) if "D" in list: dlist =(list.count("D")*10) if "N" in typelist: nlist =(list.count("N")*5) if "P" in typelist: plist =(list.count("P")*1) value = (plist + nlist + dlist+ qlist) print(value)piggyBank(["Q"])
arrow_forward
final big report project for java.
1.Problem Description
Student information management system is used to input, display student information records.
The GUI interface for inputing ia designed as follows : The top part are the student imformation used to input student information,and the bottom part are four buttonns,
each button meaning :
(2) Total : add java score and C++ score ,and display the result;
(3) Save: save student record into file named student.dat;
(4) Clear : set all fields on GUI to empty string;
(5) Close : close the win
arrow_forward
ASSIGNMENTWrite a Java program that meets these requirements.• Create a NetBeans project named LastnameAssign1. Change Lastname to your last name. For example, my project would be named NicholsonAssign1.• In the Java file, print a welcome message that includes your full name.• The program should prompt for an XML filename to write too The filename entered must end with .xml and have at least one letter before the period. If it does not, print a warning and prompt again. Keep nagging the user until a proper filename is entered.• Prompt the user for 3 integers.o The three integers represent red, blue, and green channel values for a color.o Process the numbers according to the instructions belowo Once you have handled the 3 input values, prompt the user againo Keep prompting the user for 3 integers until the user enters the single word DONEo Reading input should not stop for any reason except for when the user enters DONE.o The user will not mix DONE and the numbers. The user will either…
arrow_forward
For this assignment *c++ & no ifstream, you need to build a class that contains basic information about students and courses that have those students on the roster. You also need to build a menu that supports basic CRUD functionality for those courses. The requirements are as follows:
The student class should contain the following data about a student:
Name (dynamic char array)
Classification (char)
Major (dynamic char array)
StudentId (can be an int or a dynamic char array)
The course class should contain the following data about a course:
Course Name (dynamic char array)
Course Code (char array of size 7)
Location (char array of size 10)
Roster (dynamic student array)
The menu program should act as a driver program that manages a dynamically allocated array of courses and allows the user to:
Add a course to the list
Add students to a course
Update a course to change any of the data above including edits to students or adding/removing students from the roster
List courses…
arrow_forward
/** To change this license header, choose License Headers in Project Properties.* To change this template file, choose Tools | Templates* and open the template in the editor.*/package DataStructures;
import ADTs.StackADT;import Exceptions.EmptyCollectionException;
/**** @author Qiong* @param <T>*/public class LinkedStack<T> implements StackADT<T> {
int count;SinglyLinkedNode<T> top;public LinkedStack(){top = null;count = 0;}public LinkedStack(T data){top = new SinglyLinkedNode(data);count = 1;}@Overridepublic void push(T element) {// TODO implete the push method// The push method will insert a node with holds the given input into the top of the stack
}
@Overridepublic T pop() throws EmptyCollectionException {if (this.isEmpty()) throw new EmptyCollectionException();SinglyLinkedNode<T> node = top;top = top.getNext();count--;node.setNext(null);return node.getElement();}
@Overridepublic T peek() throws EmptyCollectionException {return null;//TODO: Implement…
arrow_forward
BELOW IS THE NETBEAN SOURCE CODE OF A SINGLETON PROJECT.
Run the code in Netbeans and provide screenshots to verify that it runs successfully.
Draw a UML Class diagram to model the program.
/** To change this license header, choose License Headers in Project Properties.* To change this template file, choose Tools | Templates* and open the template in the editor.*/package singletontest;
/**** @author lucky*/public class SingletonTest {
/*** @param args the command line arguments*/public static void main(String[] args) {for(int i=0;i<5;i++){
Singleton.getInstance();//here we can check how many instance created using below line of statemnt in loop System.out.println("singletontest.SingletonTest.main()"+Singleton.getInstance());}} }
// Double Checked Locking based Java implementation of// singleton design patternclass Singleton{ //create private instance of class for data hidingprivate volatile static Singleton obj;//private consttuctorprivate Singleton() {}//we create static…
arrow_forward
Create code in Python that produce truth tables for zyBooksFor zyBooks end of section 1.4 Exercise 1.4.5 (b,c). Use the resulting tables to determine logical equivalence as described in the text directions.
Include good, contextualized (i.e. in plain English) comments to explain each row of the truth tables, identifying any key rows used in determining logical equivalence.
Use the features in the truth table library to format the key rows visually for easy readability and comprehension
See Excercise Below
j: Sally got the job.
l: Sally was late for her interview
r: Sally updated her resume.
Express each pair of sentences using logical expressions. Then prove whether the two expressions are logically equivalent.
(a) If Sally did not get the job, then she was late for her interview or did not update her resume.
If Sally updated her resume and did not get the job, then she was late for her interview.
(b) If Sally did not get the job, then she was late for her interview…
arrow_forward
In python, complete both sections please
Section 5: The StudentAccount class This class represents a financial status of the student based on enrollment and is saved to a Student object as an attribute. This class should also contain an attribute that stores the price per credit, initially $1000/credit. This cost can change at any time and should affect the future price of enrollment for ALL students. Attributes Type Name Description Student student The Student object that owns this StudentAccount. numerical balance The balance that the student has to pay. dict loans A dictionary that stores Loan objects accessible by their loan_id. Methods Type Name Description numerical makePayment(self, amount) Makes a payment towards the balance. numerical chargeAccount(self, amount) Adds an amount towards the balance. Special methods Type Name Description str __str__(self) Returns a formatted summary of the loan as a string. str __repr__(self) Returns the same formatted summary as __str__.…
arrow_forward
BELOW IS THE NETBEAN SOURCE CODE OF A SINGLETON PROJECT.
Run the code in Netbeans and provide screenshots to verify that it runs successfully.
Draw a UML Class diagram to model the program.
/** To change this license header, choose License Headers in Project Properties.* To change this template file, choose Tools | Templates* and open the template in the editor.*/package singletontest;
/**** @author Dsquared*/public class SingletonTest {
/*** @param args the command line arguments*/public static void main(String[] args) {for(int i=0;i<5;i++){
Singleton.getInstance();//here we can check how many instance created using below line of statemnt in loop System.out.println("singletontest.SingletonTest.main()"+Singleton.getInstance());}} }
// Double Checked Locking based Java implementation of// singleton design patternclass Singleton{ //create private instance of class for data hidingprivate volatile static Singleton obj;//private consttuctorprivate Singleton() {}//we create static…
arrow_forward
In python. Please do not copy elsewhere as it is all incorrect, and include docstring so that I can understand the information so I can apply the methods to future problems. Thanks.
import the **json** module.Write a class named SatData that reads a [JSON file containing data on 2010 SAT results for New York City](https://data.cityofnewyork.us/api/views/zt9s-n5aj/rows.json?accessType=DOWNLOAD) and writes the data to a text file in CSV format. That same JSON file will be provided as a local file in Gradescope named **sat.json**. Your code does not need to access the internet.CSV is a very simple format - just commas separating columns and newlines separating rows (see note below about commas that are part of field names). You'll see an example of CSV format below. There is a csv module for Python, but you will not use it for this project.Your class should have:* an init method that reads the file and stores it in whatever data member(s) you prefer. Any data members of the SatData class…
arrow_forward
Assignment 1 University Library SystemIn this assignment you are going to implement your own version of university library system,The system will have two different sides , the first one is to help the librarian to do his job and the other side for admin to manage every one permissions in the system , so You should provide the following features in your system: Admin viewo Add/remove Studentso Add/remove librariano Add/remove other admins Librarian viewo Add/Delete bookso Issue/Return bookso View bookso View Issued bookso Log in /log outo Search for the book(id/name) Simple backend for your system , You could use a file each row should represent item o Books File should be something like:Id , Book Name, Author Name, Available quantity, Issued Quantity 1,Oliver Twist, Charles Dickens,98,2In the previous the first row was the name of each column , you can do that orhandle it in your code , id =1 , Book Name =Oliver Twist ..Etc. Tables neededo Books Id Book Name Author Name…
arrow_forward
java program
For this question, the server contains the id, name and cgpa of some Students in a file. The client will request the server for specific data, which the server will provide to the client.
Server: The server contains the data of some Students. For each student, the server contains id, name and cgpa data in a file. Here's an example of the file:
data.txt101 Saif 3.52201 Hasan 3.81....
Create a similar file in you server side. The file should have at least 8 students.
The client will request the server to provide the details (id, name, cgpa) of the Nth highest cgpa student in the file. If N's value is 1, the server will return the details of the student with the highest cgpa. if N's value is 3, the server will return the details of the student with the third highest cgpa.
If N's value is incorrect, the server returns to the client: "Invalid request".
Client:
The client sends the server the value of N, which is an integer number. The client takes input the value of N…
arrow_forward
i need comments on the code example: int x; // declare x
and life cycle
VB Code
Imports SystemImports System.Collections.GenericImports System.ComponentModelImports System.DataImports System.DrawingImports System.LinqImports System.TextImports System.Threading.TasksImports System.Windows.Forms
Namespace StudentPublic Partial Class Form1Inherits Form
Public Sub New()InitializeComponent()End Sub
Private Sub btnCalculatePerc_Click(ByVal sender As Object, ByVal e As EventArgs)txtPercentage.Text = ((Convert.ToInt32(txtMark1.Text) + Convert.ToInt32(txtMark2.Text) + Convert.ToInt32(txtMark3.Text)) / 3).ToString()End Sub
Private Sub btnDisplayGrade_Click(ByVal sender As Object, ByVal e As EventArgs)If Convert.ToDouble(txtPercentage.Text) >= 80 ThentxtLevel.Text = "Excellent"ElseIf Convert.ToDouble(txtPercentage.Text) >= 70 AndAlso Convert.ToDouble(txtPercentage.Text) <= 79 ThentxtLevel.Text = "Very Good"ElseIf Convert.ToDouble(txtPercentage.Text) >= 60 AndAlso…
arrow_forward
Hello,
I am having an error and cannot figure out how to solve this question. Could someone please assist?
Please be advised, I am using PostgreSQL as that is what was used in class. Thus, I must use PLPGSQL
Application: PostgreSQL 12 or 13PLPGSQL
CREATE OR REPLACE FUNCTION Moreno_03_bankTriggerFunction()RETURNS TRIGGERLANGUAGE PLPGSQLAS$BODY$CREATE TRIGGER Moreno_03_bankTriggerAFTER DELETE ON accountFOR EACH ROW EXECUTE PROCEDURE Moreno_15_bankTriggerFunction();
TABLES (if needed)
CREATE TABLE branch (branch_name varchar(35),branch_city varchar(15),assets numeric(15,2) CHECK (assets > 0.00),CONSTRAINT branch_pkey PRIMARY KEY (branch_name));CREATE TABLE customer (ID varchar(15),customer_name varchar(25) NOT NULL,customer_street varchar(35),customer_city varchar(15),CONSTRAINT customer_pkey PRIMARY KEY (ID));CREATE TABLE loan (loan_number varchar(15),branch_name varchar(35),amount numeric(15,2),CONSTRAINT loan_pkey PRIMARY KEY (loan_number),CONSTRAINT loan_fkey FOREIGN KEY…
arrow_forward
I have part of this code in PHP that returns data in JSON. I NEED IT TO RETURN THE DATA IN XML. THANK YOU
<?phpinclude_once 'pelicula.php';class ApiPeliculas{
function getAll(){$pelicula = new Pelicula();$peliculas = array();$peliculas["items"] = array();
$res = $pelicula->obtenerPeliculas();
if($res->rowCount()){while ($row = $res->fetch(PDO::FETCH_ASSOC)){ $item=array("id" => $row['id'],"title" => $row['title'],"year" => $row['year'],"fresh" => $row['fresh'],"score" => $row['score']);array_push($peliculas["items"], $item);}echo json_encode($peliculas);}else{echo json_encode(array('mensaje' => 'No hay elementos'));}}}?>
arrow_forward
i need life cycle and comments on these codes
(example: int x; // declare x ; )
VB Code
Imports SystemImports System.Collections.GenericImports System.ComponentModelImports System.DataImports System.DrawingImports System.LinqImports System.TextImports System.Threading.TasksImports System.Windows.Forms
Namespace StudentPublic Partial Class Form1Inherits Form
Public Sub New()InitializeComponent()End Sub
Private Sub btnCalculatePerc_Click(ByVal sender As Object, ByVal e As EventArgs)txtPercentage.Text = ((Convert.ToInt32(txtMark1.Text) + Convert.ToInt32(txtMark2.Text) + Convert.ToInt32(txtMark3.Text)) / 3).ToString()End Sub
Private Sub btnDisplayGrade_Click(ByVal sender As Object, ByVal e As EventArgs)If Convert.ToDouble(txtPercentage.Text) >= 80 ThentxtLevel.Text = "Excellent"ElseIf Convert.ToDouble(txtPercentage.Text) >= 70 AndAlso Convert.ToDouble(txtPercentage.Text) <= 79 ThentxtLevel.Text = "Very Good"ElseIf Convert.ToDouble(txtPercentage.Text) >= 60 AndAlso…
arrow_forward
Please help step by step with R program with a final code for understanding thank you.
Market Penetration.csv:
Country Facebook PenetrationUnited States 52.56Brazil 33.09India 5.37Indonesia 19.41Mexico 32.52Turkey 41.69United Kingdom 51.61Philippines 30.12France 39.07Germany 30.62Italy 38.16Argentina 49.35Canada 53.45Colombia 40.01Thailand 27.13
arrow_forward
(In java please) ( NO TOY CLASS PLEASE READ INSTRUCTION OR DOWN VOTE PLEASE LOOK AT TWO IMAGES BELOW FIRST ONE IS CURRENCY CLASS NEED THE SECOND IS INSTRUCTIONS ON HOW TO MAKE TEST CLASS. PLEASE NO TOY CLASS OR DOWN VOTE USE CURRENCY CLASS INSTEAD AND UPDATE IT) THIS A LinkNode structure or class which will have two attributes - a data attribute, and a pointer attribute to the next node. The data attribute of the LinkNode should be a reference/pointer of the Currency class of Lab 2. Do not make it an inner class or member structure to the SinglyLinkedList class of #2 below. A SinglyLinkedList class which will be composed of three attributes - a count attribute, a LinkNode pointer/reference attribute named as and pointing to the start of the list and a LinkNode pointer/reference attribute named as and pointing to the end of the list. Since this is a class, make sure all these attributes are private. The class and attribute names for the node and linked list are the words in bold in #1…
arrow_forward
/** To change this license header, choose License Headers in Project Properties.* To change this template file, choose Tools | Templates* and open the template in the editor.*/
package Linked_List;
import java.util.Scanner;
/**** @author lab1257-15*/public class Demo {
public static boolean has42 (RefUnsortedList<Integer> t){t.reset();for (int i=1; i<=t.size();i++)if (t.getNext()==42)return true;return false;}public static void RemoveAll(RefUnsortedList<Integer> t, int v){while(t.contains(v)){t.remove(v); } }public static void main(String[] args) {}}
/** To change this license header, choose License Headers in Project Properties.* To change this template file, choose Tools | Templates* and open the template in the editor.*/
package Linked_List;/**** @author lab1257-15*/public class LLNode<T>{private LLNode<T> link;private T info;public LLNode(T info){this.info = info;link = null;}public void setInfo(T info)// Sets info of this LLNode.{this.info =…
arrow_forward
47.
What statement of the following is the most appropriate?
Group of answer choices
The node class defined in the textbook can be implemented as a template class, providing more limitation to implement other container classes.
The node class defined in the textbook can be implemented as a template class, providing more memory leak possibility to implement other container classes.
The node class defined in the textbook can be implemented as a template class, providing more flexibility to implement other container classes.
The node class defined in the textbook can be implemented as a template class, providing more tool box to implement other container classes.
The node class defined in the textbook can not be implemented as a template class.
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
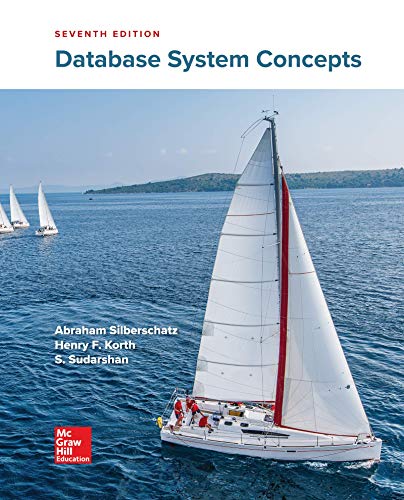
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
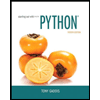
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
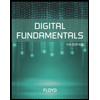
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
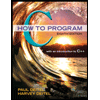
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
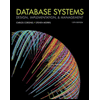
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
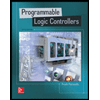
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
describe the code in detail add comment lines
package com.templatepattern;
public class TemplatePatternTest1 {
public static void main(String[] args) {String connectionString = "server=192.168.0.7";String username = "learner";String password = "designPatterns";StudentRegistration sr = new DatabaseBasedStudentRegistration(connectionString, username, password);System.out.println();Person p1 = new Person("patrick", "nick");System.out.println("Registering " + p1);sr.register(p1);System.out.println();
Person p2 = new Person("zayn", "nick");System.out.println("Registering " + p2);sr.register(p2);System.out.println();}
}
abstract class StudentRegistration {// template methodfinal Student register(Person person) {if (checkPerson(person)) {startOperation();try {int studentId = getNewIdentity(person);Student student = new Student(person, studentId);completeOperation();return student;}catch (Exception e) {cancelOperation();promptError(e.getMessage());}}else {promptError(person + " is not a valid…
arrow_forward
/** To change this license header, choose License Headers in Project Properties.* To change this template file, choose Tools | Templates* and open the template in the editor.*/package Apps;
import ADTs.QueueADT;import DataStructures.ArrayStack;import ADTs.StackADT;import DataStructures.LinkedQueue;import java.util.Scanner;
/**** @author Qiong*/public class RepeatStrings {public static void main(String[] argv) throws Exception{final int SIZE = 3;Scanner keyboard = new Scanner(System.in);QueueADT<String> stringQueue;//stringQueue = new CircularArrayQueue<String>(SIZE);stringQueue = new LinkedQueue<String>();StackADT<String> stringStack;stringStack = new ArrayStack<String>(SIZE);String line;for (int i = 0; i < SIZE; i++){System.out.print("Enter a line of text which includes only 3 words > ");line = keyboard.nextLine();//TODO enque the new element//TODO push the new element}System.out.println("\nOrder is: ");for (int i = 0; i < SIZE; i++){// TODO…
arrow_forward
1. A list of students (student ID, first name, last name, list of 4 test grades) will be transformed intoStudent objects. We will provide this file for you.a. Each feature will be separated by commas, however; the grades will be separated byspaces, as shown in this example line: 82417619,Erik,Macik,95.6 85 100 88[Hint: It seems like you might need to use the split method twice]b. Your program should work with any file with any number of lines.2. A menu will be displayed on loop to the user (meaning after a user completes a selection, themenu will be displayed again, unless the user exits).The menu options should be the following:a. View Student Grade and Averageb. Get Test Averagec. Get Top Student per examd. Exit3. Your system shall incorporate the following classes. Each class will require one file.GRADE CALCULATORPurposeA class that contains useful methods that can be used to calculate averages andconvert grades to letter gradesAttributes: NoneMethodsconvertToLetterGrade(double…
arrow_forward
A document is represented as a collection paragraphs, a paragraph is represented as a collection of sentences, a sentence is represented as a collection of words and a word is represented as a collection of lower-case ([a-z]) and upper-case ([A-Z]) English characters.
You will convert a raw text document into its component paragraphs, sentences and words. To test your results, queries will ask you to return a specific paragraph, sentence or word as described below.
Alicia is studying the C programming language at the University of Dunkirk and she represents the words, sentences, paragraphs, and documents using pointers:
A word is described by .
A sentence is described by . The words in the sentence are separated by one space (" "). The last word does not end with a space(" ").
A paragraph is described by . The sentences in the paragraph are separated by one period (".").
A document is described by . The paragraphs in the document are separated by one newline("\n"). The last paragraph…
arrow_forward
A document is represented as a collection paragraphs, a paragraph is represented as a collection of sentences, a sentence is represented as a collection of words and a word is represented as a collection of lower-case ([a-z]) and upper-case ([A-Z]) English characters.
You will convert a raw text document into its component paragraphs, sentences and words. To test your results, queries will ask you to return a specific paragraph, sentence or word as described below.
Alicia is studying the C programming language at the University of Dunkirk and she represents the words, sentences, paragraphs, and documents using pointers:
A word is described by .
A sentence is described by . The words in the sentence are separated by one space (" "). The last word does not end with a space(" ").
A paragraph is described by . The sentences in the paragraph are separated by one period (".").
A document is described by . The paragraphs in the document are separated by one newline("\n"). The last paragraph…
arrow_forward
m6 lb
Dont copy previous answers they are incorrect. Please provide a typed code and not pictures. The template was given below the code. we are just making changes to main cpp. We are writing one line of code based on the comments.
C++
use shared pointers to objects, create vectors of shared pointers to objects and pass these as parameters.
You need to write one line of code. Declare circleTwoPtr. Watch for **** in comments.
Template code is given make changes and add stuff based on comments:
Template given:
main.cpp:
#include<iostream>#include "Circle.h"#include <fstream>#include <vector>#include <memory>#include<cstdlib>#include <fstream>#include <sstream>using namespace std;const int SIZE = 10;// pay attention to this parameter listint inputData(vector<shared_ptr<Circle>> &circlePointerArray, string filename)
{ ifstream inputFile(filename); istringstream instream; string data; int count =0; int x,y,radius; try{ if…
arrow_forward
Hey, question about DLL-libraries, C#.
So I did a project where I have two classes database.cs & info.cs. I created .dll file about those in other project. Now I added .dll file to my main project to References and it's there. I'm using "using ClassMembers(dll file) in other forms now. Do I delete my two classes database.cs & info.cs now or do I keep them? This is my first time using .dll files and I'm kinda confused, what to do now..
arrow_forward
Building Additional Pages
In this lab we will start to build out the Learning Log project. We’ll build two pages that display data: a page that lists all topics and a page that shows all the entries for a particular topic. For each of these pages, we’ll specify a URL pattern, write a view function, and write a template. But before we do this, we’ll create a base template that all templates in the project can inherit from.
arrow_forward
Considering the dates API in java.time, it is correct to say:
1.
It is possible to represent a date as text in any language, as long as you provide the respective information as Locale.
B.
It is not a Postgres database compatible type.
Ç.
It is not possible to represent dates before the year 2000.
D.
The main class in this package is java.util.Date.
5.
It follows the standard defined in ISO 8601, so it is ONLY possible to display the data in English.
arrow_forward
Need help with this python question. You can't use break, str.endswith, list.index, keywords like: await, as, assert, class, except, lambda, and built in functions like: any, all, breakpoint, callable.
(Question in images at bottom)
Starter Code
import random
def spider_web(web_map, starting_place, destination):
pass
def spider_web_rec(web_map, starting_place, destination, visited):
pass
def make_spider_web(num_nodes, seed=0):
if seed:
random.seed(seed)
web_map = {}
for i in range(1, num_nodes + 1):
web_map[f'Node {i}'] = []
for i in range(1, num_nodes + 1):
sample = random.sample(list(range(i, num_nodes + 1)), random.randint(1, num_nodes - i + 1))
print('sample', i, sample)
for x in sample:
if i != x:
web_map[f'Node {i}'].append(f'Node {x}')
web_map[f'Node {x}'].append(f'Node {i}')
return web_map
if __name__ == '__main__':
num_nodes, seed = [int(x) for x…
arrow_forward
hello c++ programming
Superhero battle time! Muscles and Bones are not happy with each other and need to come to some kind of understandingWhat are the odds that Muscles will come out ahead? What about Bones?
Start with this runner file that will handle the simulation, and don't modify the file in any way (runner file is below)Spoiler time... When it's all said and done, Muscles should win about 72% of the time, and Bones about 28%
Create files TwoAttackSupe.h and TwoAttackSupe.cpp that collectively contain the following functionality:
The class name will be TwoAttackSupe
The class will be a base class for what you see below in the next blurb
The class has one member variable, an integer value that maintains a health value
Its only constructor takes one parameter, which is the health value
Make sure to set up a virtual destructor since this is a base class
Develop a function called IsDefeated() which returns a bool
Return true if the health value is less than or equal to 0
Return…
arrow_forward
You must convert this C file into C++ environments without using Classes conceptCODE: CONTACT MANAGEMENT SYSTEM#include<stdio.h>#include<conio.h>#include<string.h>#include<process.h>#include<stdlib.h>#include<dos.h>struct contact{ long ph; char name[20],add[20],email[30];} list;char query[20],name[20];FILE *fp, *ft;int i,n,ch,l,found;int main(){main: system("cls"); /* ************Main menu*********************** */ printf("\n\t **** Welcome to Contact Management System ****"); printf("\n\n\n\t\t\tMAINMENU\n\t\t=====================\n\t\t[1] Add a new Contact\n\t\t[2]List all Contacts\n\t\t[3] Search for contact\n\t\t[4] Edit aContact\n\t\t[5] Delete a Contact\n\t\t[0]Exit\n\t\t=================\n\t\t"); printf("Enter the choice:"); scanf("%d",&ch); switch(ch) { case 0: printf("\n\n\t\tAre you sure you want to exit?"); break; /* *********************Add new contacts************ */ case 1: system("cls"); fp=fopen("contact.dll","a"); for (;;) {…
arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
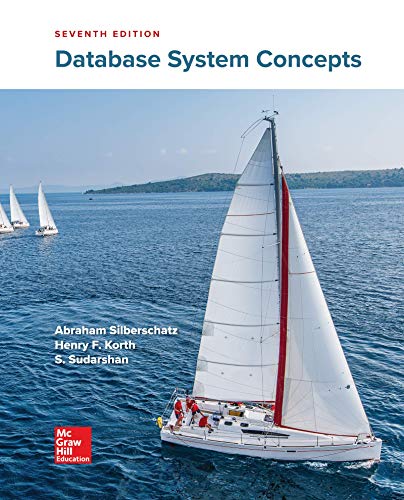
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
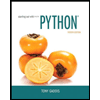
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
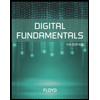
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
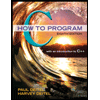
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
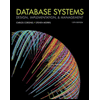
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
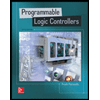
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
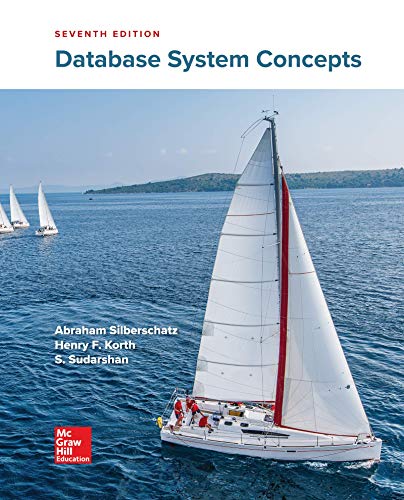
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
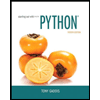
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
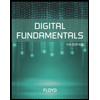
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
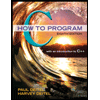
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
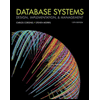
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
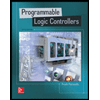
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education